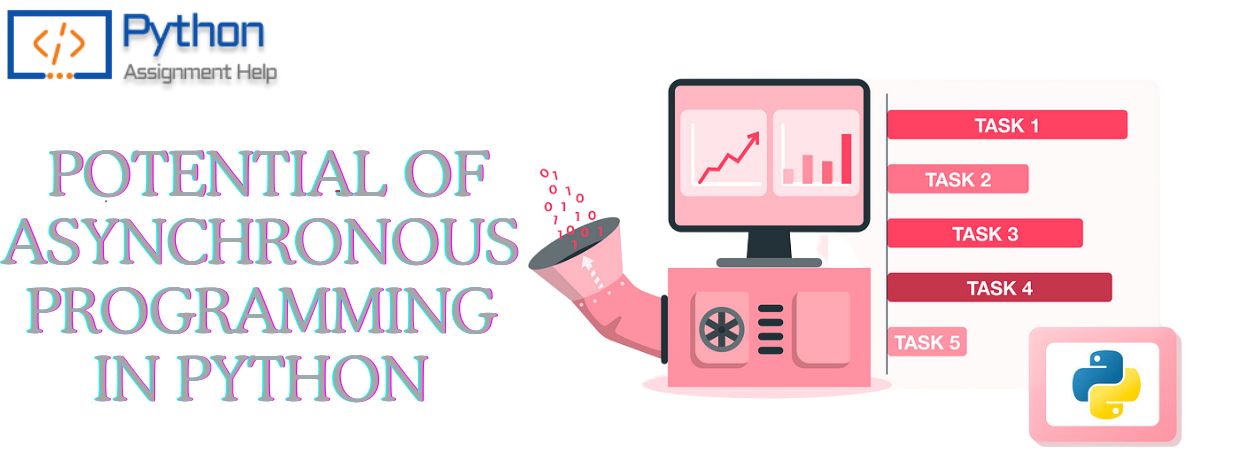
- 15th Mar 2024
- 16:26 pm
I. Unveiling Asynchronous Programming: Efficiency beyond Waiting
A. Redefining Efficiency: Asynchronous Programming in Python
Traditional synchronous programming in Python runs code line by line. This method becomes inefficient when dealing with operations that require waiting, such as network queries or user input. Asynchronous programming introduces a new paradigm, allowing your application to handle numerous activities concurrently while enhancing responsiveness and speed.
B. Asynchronous Toolbox: Key Concepts Explained
Asynchronous programming in Python requires a few essential tools:
- Async/await: This sophisticated syntax enables you to build asynchronous functions (coroutines) and control their execution. The 'async' keyword indicates that a function is asynchronous, while 'await' stops execution until a coroutine completes a waiting job (such as a network request). This allows your software to move on to other tasks while waiting.
- Coroutines: Imagine functions with superpowers! Coroutines can be paused mid-execution with 'await' and restarted later. Unlike conventional functions, they do not pause the entire application while waiting. This allows your software to handle several coroutines easily.
- Event Loops: Consider the event loop to be the conductor of an asynchronous symphony. It handles numerous coroutines, monitoring their states and switching between them as tasks are ready to resume execution. This makes better use of resources and keeps your software from becoming stuck waiting for a single job.
C. Breaking the Monotonic Chain: Asynchronous vs Synchronous
Synchronous programming works similarly to a one-lane road. The code waits for each task (such as a network request) to complete before going on to the next.
In contrast, asynchronous programming behaves similarly to a multi-lane expressway. Your application can handle numerous jobs at once, taking advantage of wait times for other processes to complete. This results in a more responsive and performant application.
In layman's words, synchronous code waits patiently, but asynchronous code continues to move on, even if it must wait for anything in the interim. This ability to manage numerous activities efficiently makes asynchronous programming essential for developing current Python applications.
II. Going Deeper: Understanding Async/Await, Coroutines, and Event Loops
A. Define Asynchronous Functions Using async/await
The 'async' and 'await' keywords are fundamental to defining and using asynchronous functions (coroutines).
Here's an example:
```python
async def fetch_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
```
This `fetch_data` function is asynchronous (`async`), and it uses `await` to pause execution until the network request completes. This allows the program to continue with other tasks while waiting for the data.
B. The Power of Coroutines: Pause and Resume Execution
Coroutines are functions that can be suspended and resumed asynchronously. When a coroutine meets a 'await' statement, it passes control to the event loop. The event loop can then go to another coroutine that is ready to run. Once the awaited task is completed, the event loop restarts the coroutine where it left off.
C. The Event Loop: Coordinating Asynchronous Tasks
The asynchronous dance is managed by the event loop, which acts as the concealed conductor. It tracks all ongoing coroutines, monitors their states, and switches between them as tasks are ready to resume execution. It prevents your program from becoming bogged down while waiting for a single task to complete.
Understanding these fundamental notions will prepare you to take use of asynchronous programming in your Python projects.
III. Unlocking the Potential: Use Cases for Asynchronous Programming
A. Mastering I/O-Bound Tasks Efficiently
Asynchronous programming shines when dealing with processes that need waiting for external resources, like:
- File I/O: Large files can be read and written concurrently without interfering with the program's main thread.
- Database Queries: While waiting for a database response, your application can carry out other operations.
- Network Requests: Asynchronous code is particularly good at managing several network requests at the same time, which improves web application performance.
B. Develop Responsive and Scalable Network Applications
Asynchronous frameworks like 'asyncio' enable you to construct highly responsive and scalable network applications, such as:
- Web Servers: Asynchronous web servers can efficiently handle several concurrent connections, resulting in a better user experience for high-traffic websites.
- APIs: Asynchronous programming makes it easy to create responsive and performant APIs capable of handling a large number of concurrent queries.
C. Developing Interactive GUIs Using Asyncio and Async/Await
Asynchronous programming can improve graphical user interfaces (GUIs) as well.
- Responsive User Interfaces: Asynchronous code keeps your GUI responsive while waiting for network queries or database activities, keeping it from freezing. Users can continue to engage with other areas of the program without interruption.
- Background Tasks: Long-running tasks in a GUI can be done asynchronously, freeing up the main thread to handle UI updates and user interactions, resulting in a more seamless user experience.
As you can see, asynchronous programming is an effective way for developing modern and efficient Python apps. Mastering these principles and strategies will allow you to take your projects to a new level of performance and responsiveness.
IV. Applying Theory: Practical Applications
A. Asynchronous File I/O: Reading and Writing with Autopilot
Consider reading or writing to numerous files concurrently without interfering with the main program's operation. Asynchronous programming makes this possible by creating different threads for each file operation. This frees up the main thread to handle other activities while the I/O operations are running. This is especially useful for large files, as typical synchronous approaches would considerably slow down the entire program.
B. Getting Data Like a Boss: Concurrent Network Requests
Need to retrieve data from numerous web sources at once? Asynchronous programming is your champion. By making asynchronous network queries, your software can fetch data from multiple URLs at once. This drastically minimizes the total time required to collect all necessary information. As each request completes, the program processes the data without waiting for all responses before proceeding to the next request.
C. Creating Responsive GUIs with Asynchronous User Interactions
Asynchronous programming can be useful even for graphical user interfaces (GUIs). Here's an example. Consider an application with a button that initiates a data fetching operation. Traditionally, the program may stop while fetching data, inhibiting user interaction. With asynchronous programming, data retrieval occurs on a different thread, allowing the user to interact with other portions of the GUI uninterrupted. This enhances the user experience by ensuring responsiveness.
V. The Asynchronous Advantage: Three Benefits
A. Performance and responsiveness. Hand-in-Hand
Asynchronous programming excels at avoiding blocking operations, resulting in a significant performance improvement for tasks that require waiting for external resources like as network connections or file I/O. This results in a more responsive program that does not freeze while waiting for these actions to finish.
B. Resource Efficiency: Achieving More with Less
Concurrently performing asynchronous processes allows you to make better use of your system's resources. Your program doesn't get bogged down waiting for a single operation to finish before moving on to the next. This allows you to handle more requests or tasks simultaneously with fewer resources.
C. Scalability Made Simple: Building for the Future
Asynchronous code has a clearer structure, particularly when dealing with high workloads requiring multiple concurrent tasks. This cleaner structure facilitates code maintenance and allows you to extend your application to handle even more concurrent tasks in the future.
VI. Best Practices for Asynchronous Programming
A. Selecting the Right Tool for the Job
There are several asynchronous frameworks and libraries in Python, each with its own set of strengths. 'asyncio' is a popular choice for general-purpose asynchronous programming, whereas libraries like 'aiohttp' focus on making asynchronous HTTP queries. The framework that is best suited for your project is determined by its individual requirements.
B. Errors Do Not Stand a Chance: Graceful Exception Handling
As with any code, asynchronous programming can produce errors.
Implementing adequate error handling mechanisms is critical for gracefully managing exceptions and avoiding unexpected application failures. This ensures that your software remains stable and can recover from unforeseen events.
C. Monitoring and Optimization: Ensuring Your Application Runs Smoothly
Monitor your asynchronous application's performance to detect potential bottlenecks. Profiling tools can help you identify areas for optimization and verify that your code makes the most use of available resources. This proactive strategy ensures your application runs smoothly and efficiently.
By following these best practices, you may use asynchronous programming to create high-performance, responsive, and scalable Python apps.
VII. Powering the Real World: Asynchronous Programming in Practice
A. Case Studies: Putting Theory to Practice
- Web servers: Consider a high-traffic website. Traditional synchronous web servers struggle with several concurrent user requests. Asynchronous web frameworks such as 'aiohttp' and 'Sanic' excel in this circumstance. By processing requests asynchronously, these servers can handle a significantly higher volume of traffic more efficiently, resulting in faster response times and a more pleasant user experience.
- Chat Apps: Real-time chat applications necessitate ongoing contact among users. Asynchronous programming is great for managing such interactions. Libraries like 'asyncio' provide for concurrent handling of incoming and outgoing messages, resulting in seamless and responsive communication even with a high number of active users.
- Data processing pipelines: Asynchronous programming is extremely important in data processing pipelines. These pipelines can be substantially more efficient by managing data ingestion, processing, and storage asynchronously. For example, data can be downloaded concurrently from multiple sources before being processed and saved asynchronously in a database, reducing wait times.
B. Performance Enhancements and Scalability: The Asynchronous Advantage
Case studies regularly highlight the performance and scalability benefits of asynchronous programming. According to studies, web servers built with asynchronous frameworks can manage many more concurrent requests than their synchronous counterparts. Similarly, data processing pipelines can drastically shorten processing times by running activities asynchronously. Less resources are required to complete the same workload, which results in cost savings due to the enhanced performance. Asynchronous code also has a tendency to be easier to scale for future development without requiring significant architectural modifications because it is more modular.
C. Obstacles and Discoveries: The Path to Asynchronous Expertise
Asynchronous solution deployment comes with its own set of difficulties. Because asynchronous code executes non-linearly, debugging it can be more difficult. Furthermore, asynchronous application testing necessitates a thorough analysis of various execution contexts. But these difficulties are outweighed by the advantages. Using asynchronous programming gives developers important new perspectives on how to create scalable and effective applications. They get the ability to efficiently use system resources and think in terms of multiple jobs running at once.
VIII. A Look at Asynchronous Innovation in the Future
A. New Frameworks and Technologies:
Python's asynchronous programming environment is always changing. Popular web frameworks like Flask now have asynchronous support thanks to new frameworks like `Quart} (which is built on top of `asyncio`, which makes it simpler to use asynchronous features in already-existing web applications. Furthermore, data access in asynchronous applications is becoming more efficient with the use of asynchronous database libraries like `aiomysql` and `aiopg`.
B. Tools for Language Development and Features:
In order to better facilitate asynchronous programming, the Python language is constantly changing. Future developments could include integrated language capabilities for creating asynchronous functions and simplifying the management of concurrency. Furthermore, asynchronous programming-specific development tools may appear that facilitate debugging and profiling.
C. Innovation and Experimentation: Opening Up New Doors
New uses for asynchronous programming are made possible. Given the growing prevalence of Internet of Things (IoT) devices, asynchronous frameworks may be employed to effectively handle data processing and communication for these networked devices. Asynchronous techniques could potentially help large-scale machine learning algorithms by enabling faster model updates and training.
IX. Final Thoughts: A Revolutionary Approach
A. Synopsis: The Asynchronous Engine
You now have a solid understanding of fundamental ideas such as coroutines, event loops, and `async/await`. You now understand how these ideas combine to enable Python asynchronous programming. You've also witnessed its useful applications and the performance advantages it provides.
B. Continuous Research and Adoption
The concept of asynchronous programming is quite powerful and is quickly changing the way we create software. Keeping up with emerging frameworks and trends is essential if you want to use them in your Python projects efficiently. Adopting this strategy will enable you to create apps that are scalable, responsive, and high-performing, meeting the needs of the modern world.
C. A Paradigm Change: Asynchronous Futures
There is no denying that asynchronous programming has an impact on software development. Asynchronous programming is influencing the direction of software design by making it possible to handle concurrent tasks effectively and to maximize the use of available resources. In the years to come, asynchronous programming will surely be essential to developing reliable and scalable solutions as technology develops and new problems appear.