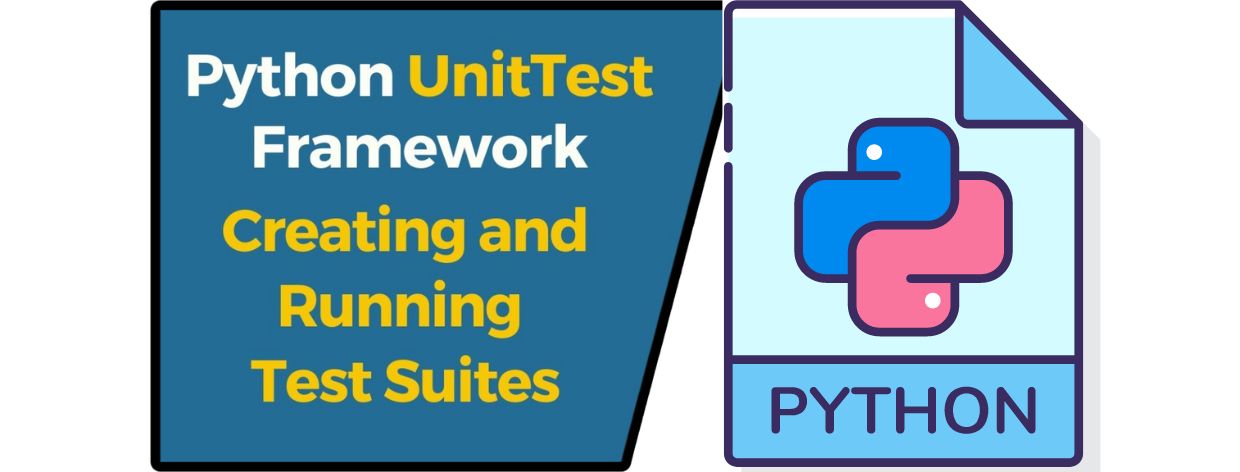
- 16th Mar 2024
- 17:20 pm
I. Introduction to Testing in Python
A. The Bedrock of Quality Software: Testing's Importance
Testing plays a crucial role in software development. It involves writing code to verify if an application behaves as expected under various conditions. As a result, bugs are found and fixed early in the development process, which leads to:
- Improved Code Quality: Testing makes sure your code works properly and follows design guidelines.
- Reduced Risks: By catching flaws early in the development lifecycle, time and resources are saved as problems are avoided.
- Enhanced Reliability: Careful testing results in programs that are less likely to malfunction in real-world settings, making them more dependable.
B. A Toolbox of Testing Frameworks:
A wide variety of testing frameworks, each with unique advantages, are available for Python:
- Unittest: The foundation for creating unit tests is provided by this built-in framework.
- It has the following features: Test cases are arranged into classes that inherit from `unittest.TestCase`.
Expectation-verifying assertions (e.g., `assertEqual`, `assertTrue`).
Test discovery, which locates and executes each test case in a directory automatically.
- pytest: A well-liked third-party framework with strong functionality and adaptability:
- Fixtures: Permit cross-test sharing of setup and takedown tasks.
- Parametrization: Allows the same test to be conducted using various data sets. understandable error messages and a rich assertion syntax.
C. Building Confidence Through Testing:
By diligently implementing testing practices, you can gain confidence in your code's quality and reliability. A well-tested codebase is easier to maintain, modify, and extend in the future, reducing the chances of introducing regressions.
II. Exploring Testing Frameworks in Depth
A. Mastering unittest: Features and Functionalities
The `unittest` framework offers a structured approach to writing unit tests:
- Test Cases: Unit tests are defined as methods within a class inheriting from `unittest.TestCase`. These methods typically start with `test_` (e.g., `test_add_function`).
- Assertions: These methods verify expected results using assertions like `assertEqual`, `assertTrue`, and `assertRaises`. Each assertion evaluates a condition and raises an error if it fails.
- Test Discovery: `unittest` automatically discovers all test cases within a directory using the `unittest.discover()` function. This simplifies running all tests without manually listing them.
B. Uncovering Pytest's Power:
A more condensed and adaptable method for writing tests is provided by pytest:
- Corrections: These functions offer reusable setup and teardown logic for tests and are decorated with `@pytest.fixture`. They enhance test isolation and aid in the management of shared resources.
- Parametrization: By enabling the same test to be performed with several sets of input data, the `@pytest.mark.parametrize` decorator reduces code duplication and increases test coverage.
- Comprehensive Assertions: Pytest has an extensive assertion syntax that includes integrated matchers and thorough error messages. This improves readability and assists in locating assertion errors precisely.
C. Unittest vs. Pytest: Selecting the Appropriate Tool for the job
Each framework has advantages and disadvantages.
- Unittest: Perfect for novices or tasks needing a methodical approach. It fits in well with conventional testing procedures and is easier to learn.
- pytest: Favored for its readability-focused design, sophisticated features, and adaptability. For larger applications or developers that want a more condensed syntax, it's a decent option.
he final decision is based on the team's preferences, the project's needs, and the preferred testing methodology.
III. Crafting a Comprehensive Testing Strategy
A. Unit Testing: The Building Blocks
Verifying the functionality of discrete code units—typically functions or modules—in isolation is the main goal of unit testing. This guarantees that every unit responds as anticipated to particular inputs.
B. Cohesion-Ensuring Integration Testing
The interaction between various modules or units is the main focus of integration testing. In order to guarantee smooth application behavior overall, it checks data flow and communication between components.
C. Simulating User Experience Through End-to-End Testing
End-to-end testing tests the complete application flow from beginning to end in order to replicate the user experience. It confirms that every part functions as planned to provide the user with the desired functionality.
Unit, integration, and end-to-end testing can all be used to obtain thorough test coverage and create a dependable Python application.
IV. Crafting Effective Test Cases: A Guide to Quality
A. Organizing Your Test Suite: Structure and Clarity
- Organization of the Test Suite: Sort related test cases into classes or modules that make sense. This makes your test suite easier to read and maintain.
- Name of Test Case: Make sure the names you choose accurately represent the functionality that is being tested. Take `test_api_returns_valid_data` or `test_add_function_positive_numbers`, for instance.
- Test Independence: Design tests so they are independent of each other's results. This isolates errors and guarantees that every test can be executed independently.
B. Crafting Sustainably Designed Tests
- Unambiguous and Brief: Make sure your tests are simple enough for other developers to comprehend as well as for you. Steer clear of test methods with nested assertions or complicated reasoning.
- DRY (Don't Repeat Yourself): To prevent repeating code in different tests, use fixtures or auxiliary functions. This lowers the complexity of the test suite and encourages maintainability.
- Focus on Behavior: Rather than concentrating on implementation specifics, test cases should confirm the expected behavior of your code. As a result, you may let your tests adjust to changes in the code without constantly updating them.
C. Adaptable Testing Using Mocks, Fixtures, and Parameterization
- Corrections: Fixtures are useful for setting up and taking down resources required for many tests, particularly in pytest. This facilitates test execution and helps manage shared resources.
- Sarcasm: Mocking libraries are useful for simulating the behavior of external dependencies (databases, APIs) during testing, as they eliminate the need for real external calls. This makes test configuration easier and isolates the code that is being tested.
- Parametrization: Use parametrization, which is supported by Pytest, to execute the same test using several data sets. As a result, there is less code duplication and better test coverage across a range of scenarios.
By following these practices, you can write effective, maintainable test cases that contribute to a robust and reliable Python application.
V. Unit Testing in Action: Putting Theory into Practice
A. Unit Testing Examples: unittest vs. pytest
unittest example: Testing a simple function for adding two numbers:
```python
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
result = add(5, 3)
self.assertEqual(result, 8)
def test_add_negative_numbers(self):
result = add(-2, -7)
self.assertEqual(result, -9)
```
pytest example (including parametrization):
```python
@pytest.mark.parametrize("x, y, expected", [(5, 3, 8), (-2, -7, -9)])
def test_add(x, y, expected):
result = add(x, y)
assert result == expected
```
B. Testing Robustness and Edge Cases:
- Criminal Situations: Provide test cases for scenarios that fall outside the scope of what can be done with your code. Try adding very big or little amounts, null values, or empty strings, for instance.
- Information Errors: To ensure that your code handles mistakes graciously, create test cases. Check for unexpected exceptions, erroneous inputs, and (if any) network outages.
- Limited States: Test input or output values near the boundaries of the predicted ranges. Test the computations, for instance, using values at the lowest and highest permitted bounds.
C. Using Code Coverage Tools to Measure Test Coverage
To find out what proportion of your codebase is covered by tests, use code coverage tools such as `coverage` or `pytest-cov`. To determine what needs more testing for thorough validation, concentrate on expanding test coverage.
VI. Integration Testing: Ensuring Interoperability
A. Testing the Interplay:
The interaction between various modules, services, and external dependencies is the main emphasis of integration testing. It guarantees that information moves between parts without hiccups and that the program functions as intended overall.
B. Simulating External Requirements:
Mocking libraries can be used to simulate replies from external services or APIs during testing. This makes it unnecessary to make actual external calls, makes test preparation easier, and guarantees reliable testing outcomes.
C. Efficiency-Boosting Automation:
Use continuous integration (CI) pipelines or tools like `pytest` to automate your integration tests. This enables you to detect integration problems early in the development lifecycle and conduct tests on a regular basis.
By using these techniques, you may thoroughly test the interactions between the various components of your Python application, resulting in a solid and dependable software foundation.
VII. User Journey Simulation via End-to-End Testing
End-to-end testing mimics real user interactions and use cases in an all-encompassing manner. The goal of these tests is to verify every step of the application process, from the first user input to the last system answer. Here's how to create end-to-end tests that work:
- Workflow Mapping: Make a detailed map of the whole user experience, noting all significant interactions and data flows between different parts. This guarantees that all of the application's crucial functionality are covered in your tests.
- Scenarios from Real Life: Create test cases that accurately depict actual user situations. Try, for instance, logging in, looking up particular information, and carrying out essential tasks related to the application's goal.
- Using Testing Instruments: For testing web applications, use frameworks like Selenium or Cypress; for testing mobile applications, use libraries like Appium. End-to-end test execution is made simpler by these technologies, which automate user actions and browser interactions.
VIII. Automating the Process for Continuous Integration and Testing
Testing is automated throughout the software development life cycle by use of continuous integration (CI) pipelines. Including testing in your continuous integration workflow has several advantages.
- Automated Testing: After a code change is committed to the version control system, continuous integration (CI) pipelines can be set up to automatically execute all unit, integration, and possibly end-to-end tests. This gives quick feedback on how modifications to the code will affect things.
- Workflows for CI/CD: Continuous delivery (CD) pipelines and continuous integration (CI) pipelines can work together to automate the deployment of code updates when they pass all tests. The process of development and deployment is streamlined as a result.
- Failure Handling and Monitoring: CI pipelines offer transparent access to test outcomes. Deployments can be stopped or alerts sent when something goes wrong, enabling developers to fix problems quickly.
IX. Encouraging a Testing Culture in Python Projects
Writing test cases is not the only step towards effective testing. The following are recommended techniques to help your development team create a robust testing culture:
- Testing as a Priority: Stress the value of testing right from the start of the project's development. Instead of being an afterthought, testing ought to be a crucial component of each development cycle.
- Documented Test Examples: As the code is updated, encourage developers to update and document their test cases. This increases the readability of the code, makes code reviews easier, and guarantees that all tests are covered.
- Collaboration and Learning: Establish a cooperative setting where developers may exchange testing expertise and benefit from one another's experiences. Code coverage and testing procedures may be the main topics of discussion during code review meetings.
Python projects can gain from better code quality, fewer regression risks, and ultimately, a more dependable and resilient application, by building a testing culture and incorporating testing into the development workflow.
X. Conclusion
A. A Python Developer's Testing Arsenal
We have explored the features and benefits of several testing frameworks, including `unittest` and `pytest`, during our investigation. We have examined various testing approaches, such as end-to-end, integration, and unit testing, and comprehended their functions in verifying various facets of an application. Best techniques for creating efficient test cases, utilizing fixtures and mocks, and calculating test coverage have also been covered.
B. Accepting Testing as a Fundamental Aspect of Quality
Writing stable and dependable Python programs requires testing, which is not just an add-on. Embracing a testing culture can help you find and repair defects early in the development cycle, write much better code, and make sure your application works as intended in a variety of scenarios.
C. Developing Trust via Extensive Testing
Investing time and effort in crafting comprehensive test suites ultimately pays off.One can feel confident in the functionality and maintainability of a well-tested codebase. It makes it easier for developers to add to and change the program, which lowers the possibility of regressions and ensures the longevity of your Python project. Always keep in mind that thorough testing is an investment in the future—a future in which your Python application prospers and provides outstanding value.